Working with JavaScript Strings: Finding a Substring in a String 5/12
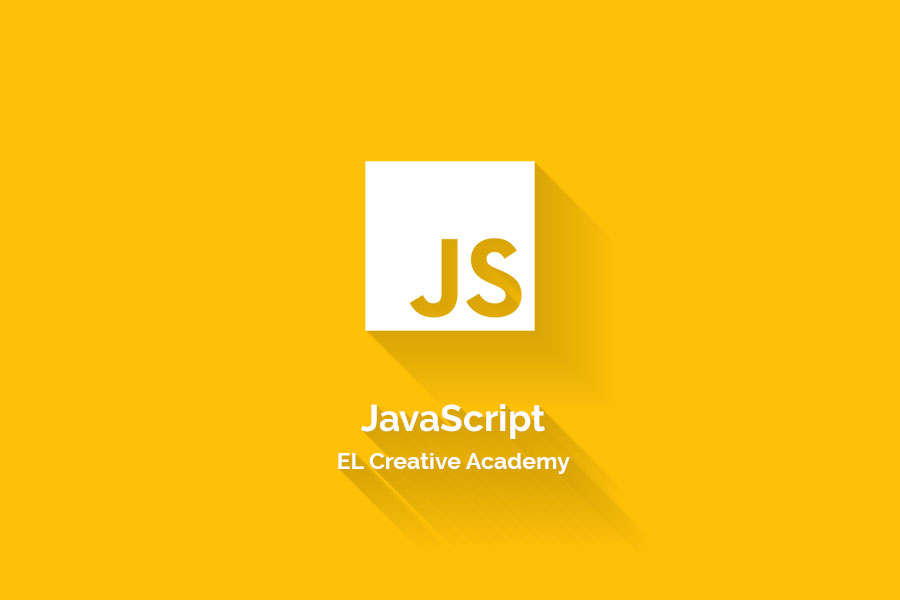
Problem You want to find out if a substring, a particular series of characters, exists in a string. Table of Contents [ Hide ] Solution Use the String object’s built-in indexOf method to find the position of the substring, if it exists: var testValue = "This is the Cookbook's test string"; var subsValue = "Cookbook"; var iValue = testValue.indexOf(subsValue); // returns value of 12, index of substringif (iValue != -1) // succeeds, because substring exists Discussion The String indexOf method returns a number representing the index , or position of the first character of the substring, with 0 being the index position of the first character in the string. To test if the substring doesn’t exist, you can compare the returned value to –1, which is the value returned if the substring isn’t found: if (iValue != -1) // true if substring found The indexOf method takes two parameters: the substring, and an optional second pa-rameter, an