Working with JavaScript Strings: Concatenating Two or More Strings 2/12
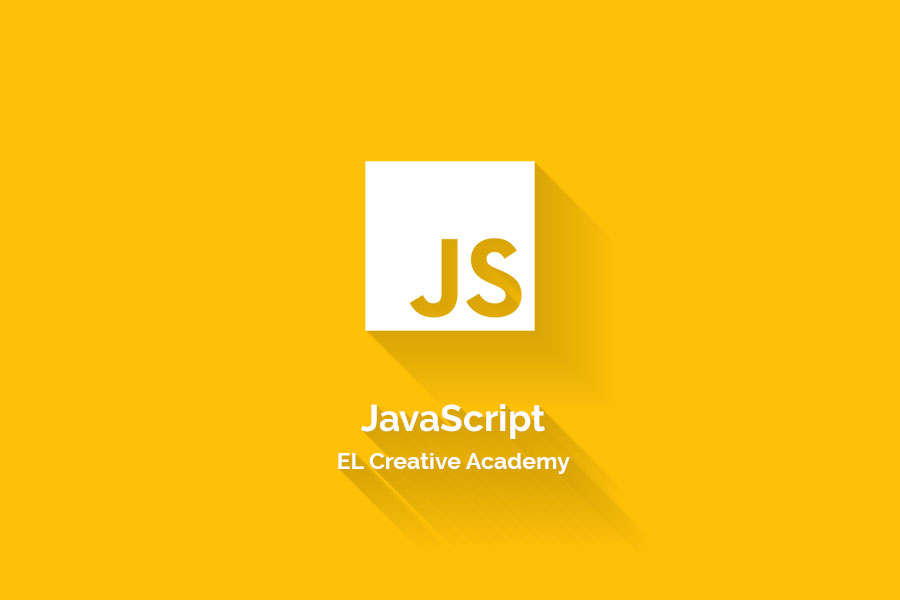
Problem
You want to merge two or more strings into one.Solution
Concatenate the strings using the addition (+) operator:
var string1 = "This is a ";
var string2 = "test";
var string3 = string1 + string2; // creates a new string with "This is a test"
Discussion
The addition operator (+) is typically used to add numbers together:
var newValue = 1 + 3; // result is 4
In JavaScript, though, the addition operator is overloaded, which means it can be usedfor multiple data types, including strings. When used with strings, the results are con-catenated, with the strings later in the equation appended to the end of the string result.
You can add two strings:
var string3 = string1 + string2;
or you can add multiple strings:
var string1 = "This";
var string2 = "is";
var string3 = "a";
var string4 = "test";
var stringResult = string1 + " " + string2 + " " + string3 + " " + string4; // result is "This is a test"
There is a shortcut to concatenating strings, and that’s the JavaScript shorthand as-signment operator (+=). The following code snippet, which uses this operator:
var oldValue = "apples";
oldValue += " and oranges"; // string now has "apples and oranges"
is equivalent to:
var oldValue = "apples";
oldValue = oldValue + " and oranges";
The shorthand assignment operator works with strings by concatenating the string onthe right side of the operator to the end of the string on the left.
There is a built-in String method that can concatenate multiple strings: concat. It takesone or more string parameters, each of which are appended to the end of the stringobject:
var nwStrng = "".concat("This ","is ","a ","string"); // returns "This is a string"
The concat method can be a simpler way to generate a string from multiple values, suchas generating a string from several form fields. However, the use of the addition operatoris the more commonly used approach.
Comments
Post a Comment